C# variables types, how to use them
In the world of programming, variables play a crucial role in storing and manipulating data. When it comes to C#, one of the most popular and powerful programming languages, understanding the different variable types and knowing how to use them is essential for writing efficient and robust code.
C# provides a rich set of variable types that cater to various data requirements. Each type has its own unique characteristics, usage scenarios, and limitations. From fundamental types like integers and floating-point numbers to more complex types like strings and arrays, the versatility of C# variable types empowers developers to build dynamic applications that handle diverse data sets.
In this article, we will delve into the world of C# variable types, exploring their definitions, applications, and best practices for utilizing them effectively in your code. Whether you are a beginner seeking a solid foundation or an experienced developer looking to reinforce your knowledge, this comprehensive guide will help you grasp the nuances of C# variable types and enable you to write code that is efficient, maintainable, and scalable.
So, if you are ready to unlock the power of C# variables and elevate your programming skills, let’s dive into the fascinating world of C# variable types and learn how to make the most of them in your code.
In any application, we can find lots and lots of variables. In this article, we will cover:
- What are variables
- What are they used for
- Different types of variables in C#
What Is a Variable?
A variable is a named place in memory reserved for your use. The amount of memory reserved for you depend on the type of the variable. The name “variables” came from the fact that the values saved in memory change (vary) throughout execution.
What identifies a variable: a name, a type, and a value. The name is the way we identify the variable (eg. color). Based on the name of the variable we can retrieve it from memory. The type of the variable, the type of information stored in it (eg. int, string, double, etc.). Last but not least we have the value, which holds the actual information (eg. red color).
The variables provide means for
- storing information
- retrieving the stored information
- modifying the stored information
The bottom line is you will use variables in c# to store and process information.
5 Questions about variables you might get in an interview
Subscribe to Relaxed Coder and get an email with 5 Questions about variables you might get in an interview.
Data Types
Where do we store variables? In the operational memory of the program (in the stack) or in the dynamic memory. Hence we have two categories:
- Primitive data types such as numbers, char, and bool. These are also called value types because they store their value in the program stack.
- Reference data types such as strings, objects, and arrays. Represented by an address that is pointing to the dynamic memory storing their value.
Primitive data types (value types in C#)
Primitive data types in C# are into these types:
- Integer types – sbyte, byte, short, ushort, int, uint, long, ulong
- Real floating-point types – float, double
- Real type with decimal precision – decimal
- Boolean type – bool
- Character type – char
Let’s have a quick look over the data types mentioned above.
Integer types represent integer numbers and are sbyte, byte, short, ushort, int, uint, long, and ulong. Interesting fact, the int type is the most often used type in programming.
Real floating-point types are real numbers that we know from mathematics. The real floating-point type is a floating point and in C# is either float or double. Float is a 32-bit real floating-point type and is also known as a single precision real number. Double is a 64-bit real floating-point type and is also known as a double precision real number. The only difference between float and double is the precision. Float has 7 decimal digits of precision and occupies 32-bit. Double has 15 decimal digits of precision and occupies 64 bits. Choosing the right floating-point type is up to you and your needs. The certain thing is that you should not use float or double for financial calculations, for those we have a decimal type.
Real type with decimal precision has a decimal numeral system rather than a binary one. Decimal type does not lose accuracy when storing and processing floating-point numbers. losses from rounding are much smaller than when using binary representation. The decimal type is convenient for financial calculations (eg. calculation of taxes, revenues, interests, payments, etc.). In comparison with float and double, the decimal type retains precision for all decimal numbers it can store.
Here are some examples of what results when using a float, double or decimal type.
float floatValue = 1;
float floatResult = floatValue / 3;
Console.WriteLine(floatResult); // 0.33333334 - rounded on the 8th decimal digit
double doubleResult = floatValue / 3;
Console.WriteLine(doubleResult); // 0.3333333432674408 - incorrect
decimal decimalValue = 1;
decimal decimalResult = decimalValue / 3;
Console.WriteLine(decimalResult); // 0.3333333333333333333333333333 - not round
Boolean type in C# has the keyword bool. It has two possible values: true and false.
Character type is a single character, a 16-bit number of a Unicode table character. In C#, it has the keyword char.
Reference data types (reference types in C#)
Examples of reference data types in C#:
Strings Objects Arrays
Strings in C# have the keyword string. Strings contain sequences of characters.
Object type is a special type in C#. This is the parent of all other types in the .NET Framework. The keyword for declaring it is the object and it can take values from any other type.
Besides the value type and the reference types, we have nullable types. These are specific wrappers around the value types that allow storing data with a null value. When are we using nullable types? We are using them for storing information, which is not mandatory. There are two ways to declare a nullable variable in C#:
Nullable<int> someVariableName = null;
int? someVariableName = null;
If we take for example int type, nullable allows us to use int as a reference type and it allows it to accept normal values and the special null value.
What about string type? What would be the difference between an empty string and null? Here is a funny image to draw a picture of the difference between string empty and null.
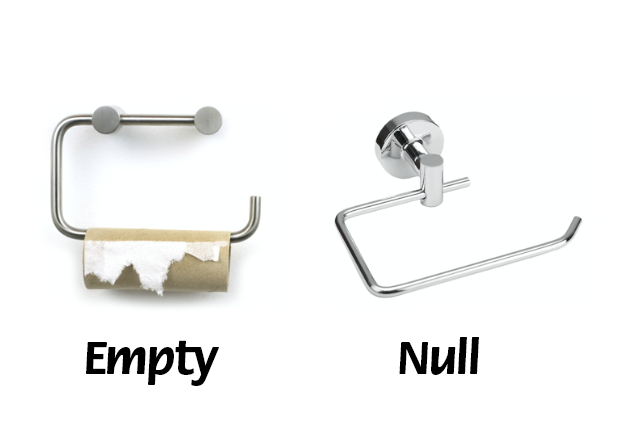
Naming variables
How you name your variables is up to you. You must follow certain rules defined in the C# language specification:
- Names can contain the letters a-z, A-Z, the digits 0-9 as well as the character ‘_’.
- The first character of the name must be a letter. The underscore is also a legal first character.
- Don’t use C# keywords as variable names (eg. base, char, default, int, object, this, null, etc.)
- Heads-up! C# is case-sensitive. Thus, for example, variables named age and Age refer to two different variables.
Besides the rules defined by the C# language specification, here are some recommendations to help you name your variables:
- Use meaningful names – the name of the variable should tell you why it exists, what it does, and how it’s used. Please be aware that if you feel the need to add a comment alongside the variable declaration. Most likely the name does not reveal the intent of the variable.
var a = 21;
var age = 21;
- Try not to use names that are different from each other in small ways. Eg. var someNameOfAnAgeVariable and someNameOfAnActVariable
- Use pronounceable names – the code you are writing should be like an open book. When part of a developers team, you will not write code for yourself. Somebody else will look over your code, he or she should be able to read it and understand it.
- Use searchable names – for variables used in many places in a block of code, is very helpful to have a name for it that is easy to search and spot. This will help you when reading the code or debugging.
I hope you found this useful. We have learned about
- What are variables
- What are they used for
- Different types of variables in C#
If you have any questions or want to say hi, please use the comments section below.