All you need to know about C# arrays and lists
The time has come to go into some details about C# arrays and C# lists. When writing code you will find that you will need to work with collections of data. We will learn about different ways of working with sequences of elements. I will explain what are arrays, how to create and initialize them, and how to iterate through them. In the second part, we will go into some details about lists. We will find out what they are, the different types of lists, and how to use them.
What is a C# array?
An array is a collection of items of the same data type stored at contiguous memory locations. You can store more variables (elements) in one array instead of declaring separate variables for each value. You can find out more about variables here . Here is a simple representation of an array containing 5 elements:
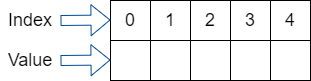
You can see in the image that the array contains two main parts: index and value. The array can contain only values of the same type (int, string, etc.). Another thing to mention is that the index of the array element always starts from zero. The index is usually used to access specific elements of an array.
Arrays can be of different dimensions. The most common ones are one-dimensional arrays and two-dimensional arrays. The one-dimensional arrays are also called vectors. The two-dimensional arrays are also called matrices.
Declaring and initializing C# arrays
C# array has a fixed length. This is set up when we initialize the array and it specifies the number of elements in an array. This is an example of how we declare a string array:
string[] textArray;
As seen above, the declaration of an array is similar to the declaration of a variable. The square bracket mark indicates that the variable is an array of elements. One thing to remember about C# arrays, they are reference-based not value-based variables. This means that when we declare an array variable, the memory is not yet allocated. The default value for our variable will be null.
When we create the C# array variable and use the new keyword, our variable’s memory will be allocated. Here is the code for initializing our array from above:
string[] textArray = new string[5];
What we have done was to initialize our string array with the length of 5 elements.
Compared to other programming languages, C# arrays have a default value. For numeric types, the default value for each element is 0. For reference types, the default value is null.
Here is an example of declaring and initializing an array in C#:
string[] monthsOfTheYear = { "January",
"February", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December" };
Starting from this example, let’s see how can we access a specific element in the array. In order to access an element of the array, we need to use the name of the array and the index of the element. Remember what we mentioned above, the indexes of the array start from zero. Here is how we would retrieve the second month of the year from our array:
Console.WriteLine($"Second month of the year is {monthsOfTheYear[1]}");
But what will happen if we want to retrieve an element located at an index that doesn’t exist?
Console.WriteLine($"Second month of the year is {monthsOfTheYear[12]}");
If you try it on your own, you will see that an exception will be thrown:
System.IndexOutOfRangeException: Index was outside the bounds of the array.
Iteration through a C# array
We have seen that retrieving a specific element from the array is straightforward. Another way of reading elements from an array is with the help of a loop. The most used loop to read arrays is the C# for loop (find out more about loops here). Here is how we can print all the months of the year with the help of the for loop:
for (int i = 0; i < monthsOfTheYear.Length; i++)
{
Console.WriteLine(monthsOfTheYear[i]);
}
The output of our code will be:
January
February
March
April
May
June
July
August
September
October
November
December
Here are two more ways of iterating an array using C# foreach and C# while. You will notice that the result is the same.
// using foreach
foreach (var month in monthsOfTheYear)
{
Console.WriteLine(month);
}
// using while
var index = 0;
while (index < monthsOfTheYear.Length)
{
Console.WriteLine(monthsOfTheYear[index]);
index++;
}
Multi-dimensional arrays
So far we have talked about one-dimensional arrays. In some cases, you will need more than one dimension to solve an issue. A multi-dimensional array is an array with more than one level or dimension. The best-known multi-dimensional array is the two-dimensions array, also known as a matrix. This is how a two-dimensional array can be represented in a diagram:
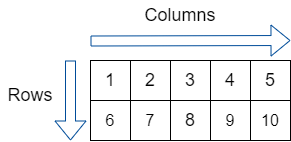
Now that we know what a C# two-dimensional array is, let’s go ahead to declare and initialize a 2D array.
int[,] matrix = {
{ 11, 12, 13, 14 },
{ 21, 22, 23, 24 },
{ 31, 32, 33, 34 }
};
This 2D array has 3 rows and 4 columns. Accessing an element of the C# 2D array is similar to getting an element from a one-dimension array. The difference is that we need to provide the indexes for both dimensions. Matrix[0,0] will return the element located on the first row and on the first column. If we want to read all the elements in our matrix we can use a C# loop. In the following example, I chose to use a C# for loop, but foreach or while can also get the job done.
int[,] matrix = {
{ 11, 12, 13, 14 },
{ 21, 22, 23, 24 },
{ 31, 32, 33, 34 }
};
for (int row = 0; row < matrix.GetLength(0); row++)
{
for (int column = 0; column < matrix.GetLength(1); column++)
{
Console.WriteLine(matrix[row, column]);
}
}
You can see that when we look for the length of each dimension we use the GetLength method and as the parameter, we use the index of the dimension.
5 Questions about arrays and lists you might get in an interview
Subscribe to Relaxed Coder and get an email with 5 Questions about arrays and lists you might get in an interview.
What is a C# list
We talked a bit about arrays, now let’s go even further and see what is the deal with lists. You might ask yourself what is a list? Well, a list is a linear data structure, an ordered sequence of elements. Lists are a good abstraction of all kinds of rows, series, sequences, and others. But we already have arrays for storing the same type of data. Why do we need lists then?
It’s better to use lists in C# when you need to do operations like sort or search because it is way more easily than in the case of arrays.
Here are the basic methods that allow us to interact with a list:
- Add(object) – adds an element at the end of the list
- Insert(int, object) – adds an element at a chosen position in the list
- Clear() – removes all elements in the list
- Contains(object) – checks whether the list contains the element
- Remove(object) – removes the element from the list
- RemoveAt(int) – removes the element at a given position (index)
- IndexOf(object) – returns the position (index) of the element
- this[int] – allows access to the elements on a set position (index)
Let’s see an example of initializing a list and using the methods mentioned above.
var animals = new List<string> { "Dog", "Cat", "Mouse" };
// Add a new animal to the list
animals.Add("Bear");
// Add snake between dog and cat
animals.Insert(1, "Sneak");
// Do we have a bear?
Console.WriteLine(animals.Contains("Bear")); // true
// Remove the bear
animals.Remove("Bear");
// Remove the snake
animals.RemoveAt(1);
// Where is the dog?
Console.WriteLine(animals.IndexOf("Dog")); // 0
// Show me the cat
Console.WriteLine(animals[1]); // Cat
// Release the animals :)
animals.Clear();
Depending on what you are developing you will use arrays, lists, or both. What you will use is up to you and the project you are working on. Regardless, I hope the information I provided to you will prove useful. Until next time! Please use the comments section below and let me know if you have any questions.