Everything you need to know about C# loops
In this post, we will talk about what tools we have at our disposal for executing code repeatedly. We will find out what a loop is and we will talk about different types of loops in C#. Among the C# loops that we will cover in this article, we find the while loop, do while, C# for, and C# foreach.
What is a loop?
While developing new applications, you will need to execute code snippets repeatedly. For this purpose, we have C# loops. The execution of the code will repeat a fixed number of times or until the given condition is true. Having this in mind you should know that there are infinite loops. These are loops that never end. Below we will go into some details on the type of C# loops.
While loop
This is one of the simplest and most commonly used loops. It composes of a condition and a body. The while loop will execute as long as the given condition is true. The condition is any expression that returns a boolean result. Two curly brackets mark the body of the loop. Here is a representation of a while loop:
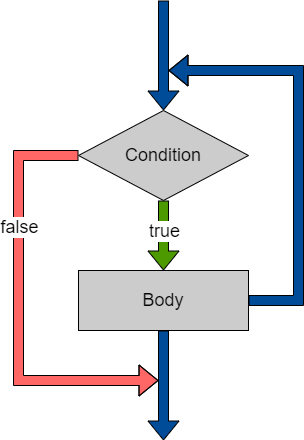
Try to follow the execution thread (blue color) in the diagram above. You can see that it evaluates the condition and if it is true, we execute the body of the C# while loop. After this, the execution thread checks the condition again. If it is true again, the program will execute the body of the while loop. All this process repeats again and again until the condition is false. When the C# while loop condition is false, the loop stops, and the execution thread continues to the first line after the while loop. Bear in mind that if the while condition is false from the beginning, the body of the while loop will not execute even once.
Here is an example of a while loop that prints the even numbers between 0 and 10:
var number = 0;
while (number < 10)
{
if (number % 2 == 0)
{
Console.WriteLine($"Number {number} is even");
}
number++;
}
And here are the results of the code from above:
Number 0 is even
Number 2 is even
Number 4 is even
Number 6 is even
Number 8 is even
There are some operators used in C# loops. One of them is the break operator. This operator exits the loop prematurely before it has completed the execution. When the execution thread reaches the break operator, the while loop terminates. Then, the execution thread executes from the first line after the while loop body ends. Here is what is happening if we apply the brake operator to our example from above:
var number = 0;
while (number < 10)
{
if (number % 2 == 0)
{
Console.WriteLine($"Number {number} is even");
break;
}
number++;
}
Output:
Number 0 is even
Do while loops
The do-while loop is very similar to the C# while loop, but it checks the condition after it executed the body. This is a loop with a condition at the end. Here is a representation of the C# do-while loop:
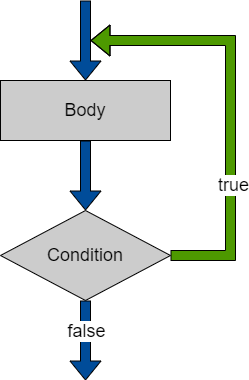
You can see in the diagram that the body of the loop executes first. After that, the program checks the condition. If the condition is true, the body of the loop executes. This logic repeats until the condition is false. When the condition is false, the loop terminates and the execution thread continues outside the loop.
Use C# do-while loop especially when you need to execute the sequence of code from the body at least once. Here is an example of how to use a do-while loop in C#:
var number = 1;
var sumOfNumbers = 0;
do
{
Console.WriteLine($"Adding number {number} to total of {sumOfNumbers}");
sumOfNumbers += number;
number++;
}
while (sumOfNumbers < 10);
Console.WriteLine($"Total {sumOfNumbers}");
5 Questions about loops you might get in an interview
Subscribe to Relaxed Coder and get an email with 5 Questions about loops you might get in an interview.
For loops
The for loop is the most common loop in C#. This loop is slightly more complicated than the other two loops but it can solve more complicated tasks with less code. It consists of four parts:
- Initialization block – executes only once, before starting the loop. In this block, we declare one or more variables. Usually, here we declare our counter variable and set its initial value. You can use this variable only inside the loop.
- Condition – the program evaluates the condition each time, similar to the while loop. When the result of the condition is true, the C# for the loop body executes. When the result is false, the loop spikes to the end, and the execution thread continues from the first line after the for loop body ends.
- Update – this part of the for loop executes each time after the body of the for loop has finished executing. Usually, here we find the update of the counter variable.
- Body – this contains the block of code that executes when the condition of the for loop is true.
This is how the for loop looks in C#:
for (initialization; condition; update)
{
body
}
And here is a diagram of how C# for loop works:
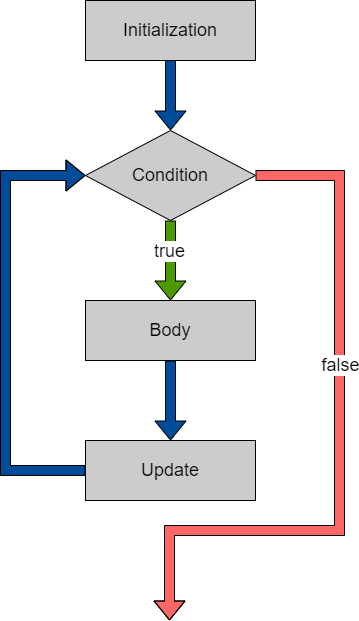
And here is what our while loop example looks like when using for loop:
for (int number = 0; number < 10; number++)
{
if (number % 2 == 0)
{
Console.WriteLine($"Number {number} is even");
}
}
If you will try the code yourself you will see that the result is the same as in the case of the C# while loop.
When talking about the while loop, I mentioned that there are some operators that are usable in C# loops. One of them was the break operator. Another one is the continue operator.
When you don’t want to execute all the code inside one iteration of the loop use the continue operator. This stops the iteration of the loop, without executing the code found after it in the loop body. The continue operator is usable in any of the C# loops mentioned in this article. Here is an example of getting all the even numbers found between 0 and 10 and not divisible by 4:
for (int number = 0; number < 10; number++)
{
if (number % 4 == 0)
{
continue;
}
if (number % 2 == 0)
{
Console.WriteLine($"Number {number} is even");
}
}
In the console, we will see this:
Number 2 is even
Number 6 is even
Foreach loops
The foreach loop is an extension of the C# for loop. This allows us to iterate over elements of a collection of elements (array, list, etc.). It is simpler than the for loop and is the preferred way because it saves writing more code. Here is the example used in the while and for loop:
int[] numbers = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
foreach (var number in numbers)
{
if (number % 2 == 0)
{
Console.WriteLine($"Number {number} is even");
}
}
When we have to process complex code we can further improve the performance of the foreach loop and use parallel foreach syntax. Compared to the foreach loop, the parallel one has a few benefits:
- Execution takes place in parallel – iterations inside the foreach loop will execute in parallel, not one by one
- Using multiple threads – the foreach loop executes on a single thread. Parallel executes on different threads, thus using the computer resources more efficiently.
- Slow processes execute faster
When choosing between foreach and parallel foreach, you should have some things in mind. Complex (slow) code will execute faster in parallel foreach. At the same time, simple (quick) code will execute slower in parallel than it would in the normal foreach loop. If it is important to process array/list items one by one in a specific order don’t use parallel foreach. Parallel foreach will not process the items in the same order as they are in the array/list.
Nested loops
The C# nested loops are consisting of multiple loops located inside each other. Here is an example of a C# for a nested loop:
for (int number = 1; number <= 3; number++)
{
for (int numberB = 1; numberB <= 3; numberB++)
{
Console.WriteLine($"Sum of {number} and {numberB} is {number + numberB}");
}
}
Console:
Sum of 1 and 1 is 2
Sum of 1 and 2 is 3
Sum of 1 and 3 is 4
Sum of 2 and 1 is 3
Sum of 2 and 2 is 4
Sum of 2 and 3 is 5
Sum of 3 and 1 is 4
Sum of 3 and 2 is 5
Sum of 3 and 3 is 6
Nested loops can be useful in some cases but you should be careful about the impact on performance. A great way of measuring the complexity of code is Big O. You can find some interesting insights on Big O here and why you should avoid using nested loops.
What do you think? What are your thoughts on loops? Head over to the comments section and share your thoughts!